Flutter Streams: A Comprehensive Guide
Posted on Mer, 14 Ago, 2024 by
Nome - 6 min read
FLUTTER
ASYNC
STREAM
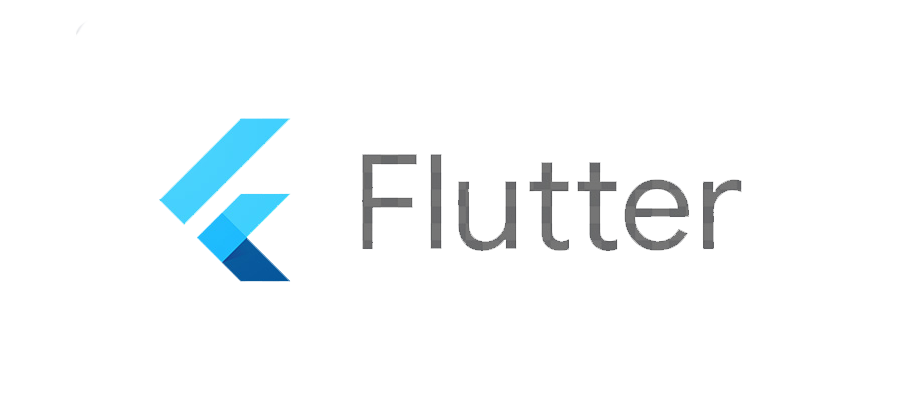
Understanding the Backbone of Asynchronous Programming
Flutter streams are a cornerstone of building reactive and efficient applications. They provide a powerful mechanism for handling asynchronous data, enabling developers to create responsive and dynamic user interfaces. In this article, we'll explore the fundamentals of streams, their practical applications, and best practices.
What are Flutter Streams?
A stream is essentially a sequence of asynchronous events. It's like a pipeline where data flows continuously. Flutter leverages streams extensively to manage various asynchronous operations, including:
- Network requests: Fetching data from APIs
- User input: Handling keyboard, mouse, or touch events
- Animations: Creating smooth and interactive animations
- State management: Managing application state in a reactive manner
Key Concepts
- StreamController: This class manages the flow of data in a stream. It allows you to add, listen, and control the stream's lifecycle.
- StreamBuilder: A widget that automatically rebuilds based on the data emitted by a stream. It's ideal for displaying dynamic content.
- StreamSubscription: Represents a listener to a stream. You can subscribe to a stream to receive data updates.
Practical Applications
- Real-time data updates: Displaying live stock quotes, chat messages, or sensor data.
- User input handling: Creating interactive forms or games that respond to user actions.
- File uploads and downloads: Tracking progress and providing feedback to the user.
- Error handling: Gracefully handling errors that occur within a stream.
Best Practices
- Efficiently manage subscriptions: Avoid unnecessary subscriptions to prevent memory leaks.
- Handle errors gracefully: Implement proper error handling to ensure app stability.
- Consider stream transformations: Use operators like
map
,where
, andasyncMap
to transform data before consumption. - Leverage StreamBuilder for UI updates: Simplify UI management by using StreamBuilder.
Advanced Topics
- Broadcast streams: Share data with multiple listeners.
- Async generators: Create custom streams using
async*
functions. - Stream chaining: Combine multiple streams for complex data processing.
By mastering Flutter streams, you'll unlock the full potential of asynchronous programming and build exceptional user experiences.