Understanding and Using Angular Injectors: The Backbone of Dependency Injection
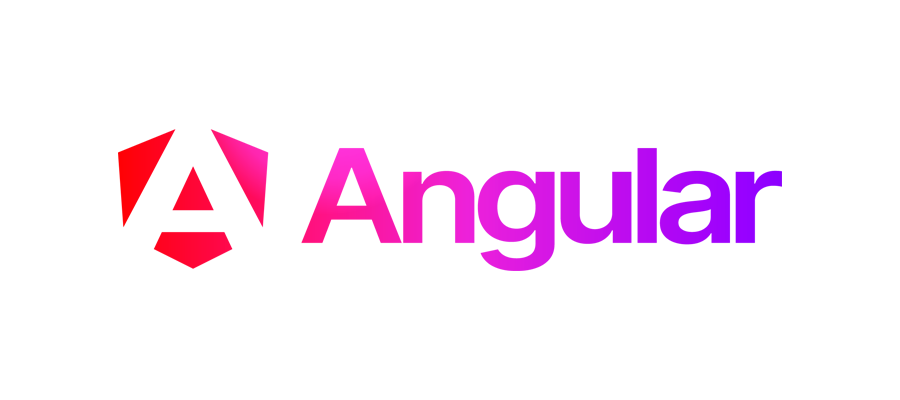
Angular's dependency injection (DI) system is a powerful tool that promotes loose coupling and code reusability. At the heart of this system lies the injector, a hierarchical structure responsible for providing dependencies to various parts of your application. In this article, we'll delve deep into understanding and using Angular injectors effectively.
1. Core Concepts: Tokens, Providers, and Hierarchy
- Tokens: These act as identifiers used to request dependencies from the injector. Tokens can be classes, strings, or custom objects created using
InjectionToken
. - Providers: These define how a dependency should be created and provided. Providers are configured using the
@Injectable()
decorator and specify details like the class to be instantiated and any dependencies it requires itself. - Injector Hierarchy: Angular has a hierarchical structure of injectors. The root injector is created with the
@NgModule
decorator and serves the entire application. Child injectors can be created for components, directives, and services, inheriting from their parent injectors.
2. Resolving Dependencies: How Injectors Find What You Need
When a component, directive, or service requests a dependency through the constructor or other injection points, the injector kicks in:
- Lookup: The injector starts searching for a provider matching the requested token in the current injector hierarchy.
- Resolution: If a matching provider is found, the injector creates or retrieves an instance of the dependency based on the provider configuration.
- Inheritance: If no provider is found in the current injector, it delegates the search to its parent injector, continuing up the hierarchy until a provider is found or the root injector is reached.
3. Putting it into Practice: Using the @Inject
Decorator
The @Inject
decorator is the primary way to request dependencies in your components, directives, and services. Here's how it works:
import { Injectable, Inject } from '@angular/core';
@Injectable({
providedIn: 'root'
})
export class MyService {
constructor(@Inject(SomeDependencyToken) private dependency: SomeDependency) {}
useDependency() {
this.dependency.doSomething();
}In this example:
@Inject(SomeDependencyToken)
tells the injector to search for a provider with the tokenSomeDependencyToken
.- The retrieved dependency is then injected into the constructor as a private property.
4. Advanced Injector Concepts: Optional Dependencies and Scoping
- Optional Dependencies: Sometimes, a dependency might not always be available. To handle this, use the
@Optional
flag with@Inject
. If the injector cannot find the dependency, it injectsnull
instead. - Dependency Scoping: Providers can be configured with different scoping options using the
providedIn
property of@Injectable
. Common options include:'root'
: The dependency is a singleton available throughout the application.'component'
: The dependency is created for each component instance.'custom'
: Use a custom injector to control the scope.
5. Best Practices for Using Injectors
- Clear and Descriptive Tokens: Choose meaningful token names to improve code readability and maintainability.
- Favor Tree-Shakeable Providers: Configure providers with
providedIn
to ensure unused dependencies are not included in the final bundle. - Consider Alternatives for Simple Dependencies: For simple values or configurations, consider using dependency injection for complex scenarios only.
By understanding how injectors work and using them effectively, you can build cleaner, more maintainable, and well-structured Angular applications. Remember, Angular's DI system is a powerful tool, and mastering the injector is a key step towards becoming a proficient Angular developer.